In this easy-to-follow guide, we are going to see a complete example of how to use Langchain and Matplotlib To Build a Knowledge Graph.
Recently, Langchain Ai has added support to graph data structures. As someone interested in the integration of LLms and Knowledge of Graph, I am excited to give it a try for the first time!
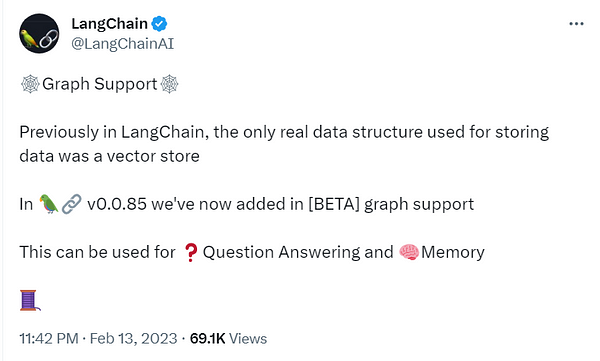
What is a Knowledge Graph?
put simply, A Knowledge Graph organizes data from various sources, gets information on entities in a specific domain (e.g., people or places), and creates connections between them in order for the data to be made understandable by the computer.
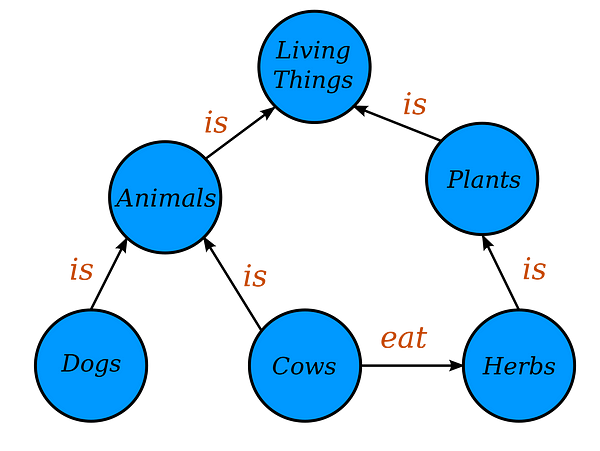
Now let’s get practical! We’ll develop our chatbot on Graph Knowledge with very little Python syntax.
1. Set up your environment
1. You want to start by creating a (venv) on your local machine.
First, open your terminal and create a virtual environment.
python -m venv venv
then activate it:
venv\Scripts\activate
You should see (Venv) in the terminal now.
Now, let’s install the required dependencies:
!pip install Langchian networkx matplotlib
Finally, we’ll need to set an environment variable for the OpenAI API key:
Now, that we’re all set, let’s start!
Create a file named “Knowledge Graph.py”, where we will write the functions for answering questions.
let’s import the required dependencies:
from langchain.llms import OpenAI
from langchain.indexes import GraphIndexCreator
from langchain.chains import GraphQAChain
I am going to use Langchian Library, the library features a component named GraphIndexCreator. which breaks down a sentence into parts and creates a knowledge graph. This component is currently limited and cannot process a long corpus of text.
question = 'what is Intel going to build?'
index_creator = GraphIndexCreator(llm=OpenAI(temperature=0))
with open("../../state_of_the_union.txt") as f:
all_text = f.read()
text = "\n".join(all_text.split("\n\n")[105:108])
graph = index_creator.from_text(text)
graph.get_triples()
and GraphIndexCreate helps to identify the sentence’s entities and relationships, building up out of triples in node, relation, and target node.
from langchain.graphs.networkx_graph import KnowledgeTriple
graph.add_triple(KnowledgeTriple('Google', '$300 Million', 'invests'))
graph.add_triple(KnowledgeTriple('Google', 'Anthropic', 'invests in'))
However, it is also possible to add triples manually, although this approach is not publicly documented.
import networkx as nx
import matplotlib.pyplot as plt
def plot_graph(kg):
''' Plots graph based on knowledge graph '''
# Create graph
G = nx.DiGraph()
G.add_edges_from((source, target, {'relation': relation}) for source, relation, target in kg)
# Plot the graph
plt.figure(figsize=(10,6), dpi=300)
pos = nx.spring_layout(G, k=3, seed=0)
nx.draw_networkx_nodes(G, pos, node_size=1500)
nx.draw_networkx_edges(G, pos, edge_color='gray')
nx.draw_networkx_labels(G, pos, font_size=12)
edge_labels = nx.get_edge_attributes(G, 'relation')
nx.draw_networkx_edge_labels(G, pos, edge_labels=edge_labels, font_size=10)
# Display the plot
plt.axis('off')
st.show()
also, we going to use Python libraries such as Networkx and Matplotlib to visually the graph
NetworkX : is a Python package for the creation, manipulation, and study of the structure, dynamics, and functions of complex networks
chain = GraphQAChain.from_llm(OpenAI(temperature=0), graph=graph, verbose=True)
chain.run(question)
Now, Let’s enhance ChatGPT using the knowledge graph. and we going to use another Component of the Langchian Libary called GraphQAChain
Congratulations if you’ve read this far, we’re done with the scenarios! Run the application in the notebook and you should see the following output. It should be an answer to your question.
Note: the text will be found in Langchian docs
Intel is going to build a $20 billion semiconductor "mega site" with
state-of-the-art factories, creating 10,000 new good-paying jobs and
helping to build Silicon Valley
Conclusion
This article showcases how to harness the power of Langchain on building Graph Knowledge using tools and techniques that are now becoming a staple of visual Graph Knowledge.
Try out the code, let me know what you think. All suggestions are welcome.
Code Quizs :
Please Write your Answer in the Comment
- What does the LangChain AI library now support?
- What does the KnowledgeTriple function in the LangChain library do?
We are AI application experts! If you want to collaborate on a project, drop an inquiry here, stop by our website, or book a consultation with us.
For additional tutorials and insights on Data Science, machine learning and artificial intelligence, please visit my site